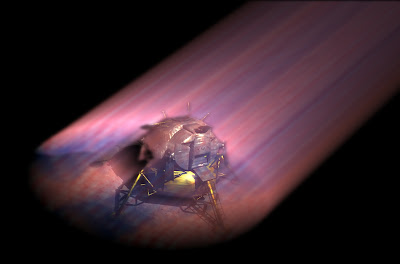
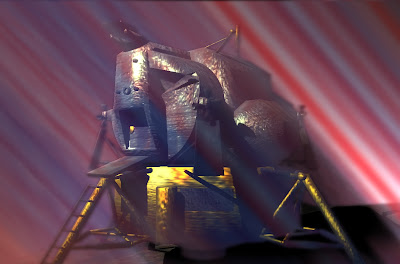
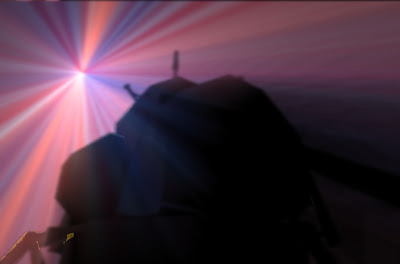
Below are screens from my deferred volumetric renderer, an attempt to render participating media or participating media renderer attempt.
It uses a simple approach for rendering volumetric fog as described in Boyd's Volumetric Rendering in Realtime at http://www.gamasutra.com/features/20011003/boyd_01.htm
or Nvidia's Fog Polygon volumes. Technique is similar to one described by Wyman And Rames in ShaderX 7 "A hybrid Method for Interactive Shadows in Homogeneous Media".
Yet it does not use any shadow volumes.
It uses naive ray marching method, but does sampling only inside desired volume.
It can render light shaft and shadow streaks in a volume as well as coloured light sources.
It does introduce aliasing and undersampling artifacts, if volume is too large.
It's uses a per pixel volume marching, but I was suprised with the results. A volume can also use a gobo as a color modifier.
Idea is simple, given world position (from deferred pass)
render as deferred light but: (in 1/4 resolution)
1. backDepth pass:
render depth of backfaces of a volume in different render target (backDepth)
(Cull CW, disable ZWrite, enable ZEnable, ZFunc always, depthStencil from deferred pass)
compare Z (depth) with Z from scene depth
if sceneDepth.z less then backDepth.z then backdepth =sceneDepth
2. frontDepth pass:
render front faces,
(Cull CCW, enable ZWrite, enable ZWrite, ZFunc lessEqual, depthStencil from deferred pass)
vector4 midPos = frontDepth - backDepth;
vector4 pointInVolume
vector4 vLightPosInFog
for(int i = 0; i less num_samples;i++)
{
pointInVolume = frontDepth - (mid_pos)*len; // traverse from backDepth to frontDepth
vLightPosInFog = transform pInVolume.xyz with matViewToLightProj; // project to light space
// inShade = tex2Dproj(ShadowMapSampler, vLightPosFrontFog).r;
// if inShade less than vLightPosInFog.z then isInShadow
if IsInShadow(pointInVolume) then shade_fog++;
}
shade_fog = shade_fog*inv_num_samples; // shadow streaks
Were len is a distance from 0.0 to 1.0;
You can either do evenly distributed sampling or jittered sampling for len.
Do the same but sample gobo texture, wihout comparison, just add texture color.
Performance on ATI Radeon 4870 is ~60 FPS.